Disclosure: Privacy Australia is community-supported. We may earn a commission when you buy a VPN through one of our links. Learn more.
21 JavaScript Interview Questions
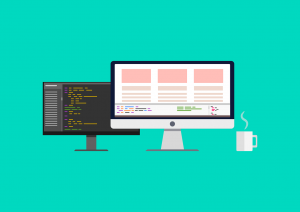
So, you are looking to hire a JavaScript developer. Or maybe you are a JavaScript developer looking to nail an upcoming interview. Whichever side of the battle lines you are on, you are probably wondering how to win.
What are the right questions to ask? How do you answer them?
This is not a simple thing to navigate. Hiring people involves evaluating personality as much as skills, and there is only so much you can know about someone through an interview. This means that on the other side of things, succeeding in an interview is also about both personality and skills.
Table of Contents:
- 21 Good Questions to Ask
- Looking at the JavaScript Developer Job as an Employer
- Looking at the JavaScript Developer Job as an Employee
- Code Challenges as an Employer
- Code Challenges as an Employee
- Conclusion
21 Good Questions to Ask
So, let’s go over 21 good questions to ask, as well as what sort of response to look for. This will also help you think about what to expect if you are going into an interview. Then, we will talk about the JavaScript developer job from both perspectives: Employer and employee.
1. Question: Why would you wrap the entire content of a JavaScript source file in a function block?
Answer: You will actually see this technique employed across a lot of different JavaScript libraries. Doing it creates a closure around all of the content in a file. It also creates a private namespace, which makes sure that you avoid clashes between namespaces of different JavaScript libraries.
2. Question: Why would you include “use strict” at the beginning of a JavaScript source file?
Answer: “Use strict” is an easy way to signal that you want to enforce stricter parsing. That means that where previously certain errors would go unflagged, they will throw exceptions.
3. Question: What is a “closure” in JavaScript?
Answer: This is an inner function, but it has access to the outer function’s scope chain. All in all, it has access to functions in three scopes: Its own scope, variables in the enclosing functions scope, variables in the enclosing function’s scope, and global variables.
4. Question: What is the difference between “undefined” and “not defined” in JavaScript?
Answer: “Not defined” and “Undefined” are both errors related to variables. But they are very different kinds of errors.
The difference is between defining and declaring. Declaring a variable means that you have stated it exists, even though you have not detailed its contents. In that case, memory will still be set aside for it. If something is not defined, then there is not even memory set aside for it.
5. Question: What are the different data types in JavaScript?
Answer: The primitive data types in JavaScript are the ones that store individual values. They are String, Number, BigInt, Boolean, Undefined, Null, and Symbol.
You also need to know of non-primitive data types, which store many and different types of values. These are not predefined in the same way that primitive data types are. Instead, they are defined by the programmer themselves.
6. Question: What is “Hoisting” in JavaScript?
Answer: Hoisting is a function built into JavaScript by default. What it does is that it moves all the variable and function declarations in a scope to the top of the scope. That scope can be local, but this also works with global scopes.
7. Question: When you delete an element of an array, does it affect its length?
Answer: No, when you delete the element of an array, it maintains the length of the array. You have only deleted its data, not the amount of memory allocated to the array.
8. Question: What is the difference between “==” and “===” operators?
Answer: These are both comparison operators. But there is a difference: “==” ensures equality between values, whereas “===” guarantees equality between both types and values.
9. Question: What is the DOM?
Answer: DOM is the acronym for Document Object Model. It allows JavaScript to manipulate HTML by creating, changing, or removing elements from the HTML.
10. Question: How do you add an element to the beginning of an array?
Answer: Use the unshift function.
11. Question: How do you add an element to the end of an array?
Answer: Use the push function.
12. Question: Imagine you have this code: “var b = [6, 7, 8, 17, 66, 2]; var c = b[12];” Will this result in a crash? Why?
Answer: Yes, it will result in a crash. The reason why is that you are attempting to access a part of the array that does not exist. By attempting to access b[12] you are attempting to access the twelfth element of the array. The variable “b” only has six elements.
These statements would throw an “indexOutOfBounds” exception.
13. Question: What would the following code return: “console.log(typeof typeof 2);”
Answer: This is an interesting one, because it executes in a different order than it appears. “typeof 2” executes first and returns “number” as a string. The first “typeof” then converts that returned value into a string.
14. Question: What is implicit type coercion in JavaScript?
Answer: It is JavaScripts way of automatically converting the value of one datatype to another. This only happens when you operate using two different data types.
15. Question: Is JavaScript a statically typed or a dynamically typed language? What does that mean?
Answer: JavaScript is dynamically typed. That means that when you declare a variable in JavaScript, you do not have to declare the type of variable.
16. Question: What are the differences between passed by value and passed by reference?
Answer: The difference comes from whether the data types are primitive or non-primitive. Primitive data types are passed by value, while non-primitive data types are passed by reference.
For something to be “passed by reference”, you are giving it an address to a value. The function will then be able to manipulate that value in memory.
When something is “passed by value”, the function receives a value. That value will not affect anything outside of the function. Any variable passed into this function is effectively copied, so that the original variable is never affected by the function.
17. Question: What is one way to write a function “isInteger(x)” that determines if “x” is an integer?
Answer: This is a good test of whether or not someone is overly reliant on ECMAscript 6, since that interpreter has a function for this sort of problem built into it. Solving this sort of problem was far more complicated before ECMAscript 6, so lacking those training wheels you would have to code it.
The best way to solve this problem without that function is a code along the lines of “function isInteger(x) {return (x ^ 0) === x;}”.
18. Question: What is the value of “typeof undefined == typeof NULL”?
Answer: This is a funny one, because despite all appearances the value will simply be “true”. The reason being that we are using NULL and not null, as JavaScript is highly case sensitive. NULL will be treated just like any other variable that goes undefined.
19. Question: What is an Immediately Invoked Function in JavaScript?
Answer: An Immediately Invoked Function is a function that runs the moment that it is defined.
20. Question: What are higher order functions in JavaScript?
Answer: Higher order functions take functions as parameters. That means they take functions as variables and manipulate them.
21. Question: What are the call(), apply(), and bind() methods?
Answer: They are all predefined functions in JavaScript. Let’s start with call(). This function calls a function with a certain value that is provided individually.
The apply() function does the same thing, but this time the value is provided as an array.
And finally, bind() creates a brand new function. When this function is called, it is set to the provided value.
Looking at the JavaScript Developer Job as an Employer ➡️
Let’s talk for a moment about the meaning of these questions. You might be (and probably should be) thinking really hard about what you are trying to accomplish by asking all these questions.
What should a JavaScript developer know? Should they be able to answer all of these questions perfectly? Aren’t there huge gaps of knowledge missing from these questions? There is so much to consider, but you really only need to think about one thing: Can they solve the problems you give them?
Asking these questions helps test this by exploring what they know, how they solve problems, and how they communicate the solutions to those problems. They do not need to be an encyclopedia of JavaScript knowledge; computers serve that purpose, but computers cannot solve our problems for us.
Filling in the Gaps in These Questions
As we alluded to earlier, these questions have gaps in them. Whether or not someone knows what a call() function is might be horribly critical to the project you want to assign them to. But it also might not be and asking that question would be a massive waste of effort to have them answer that question, much less validate their answer.
So, let’s break down a potential employee’s knowledge into three categories:
- JavaScript knowledge. This means questions like “What is this function?”
- JavaScript applicability. This means questions like “What will happen with this code?”
- Personal communication. This means questions that are not really listed here, as they are your more traditional “What is a time when you faced up to a challenge?” questions.
Thinking of it in these terms will help you sort out what questions your interview needs in such a way that you can guide your focus to the skills actually relevant to your project. If your project needs a lot more personal communication than JavaScript knowledge, you can emphasize those questions first.
Looking at the JavaScript Developer Job as an Employee ➡️
As rational as we might make the process sound, the person hiring you is unlikely to have a very clear idea as to why you are being hired. This is the big difference between the experience of the employer and the employee: There is usually a person trying to translate between the two of them.
What does that mean? Well, every job that requires a developer is going to have someone at the head of it. There are two possibilities for what is going on with this person: Either they know what they are doing, or they do not. This means when they seek to hire someone for a developer job, either they know what problem that potential employee needs to solve, or they do not.
That “do, or do not” dichotomy is going to be reflected in the person conducting the interview, whether that person is a recruiter, another developer, or the head of the project itself.
How to Deal with a Recruiter
Let’s prepare you for what is essentially the worst-case scenario. That means the project looking to hire you is run by someone incompetent, and they gave little to no instructions as to what sort of problems the recruiter should look for in your ability to solve.
That means they will ask you a lot of general knowledge questions about JavaScript. You have to come prepared with knowledge of the most common problems, including incorrect references to values, thinking in a scope that is common in other programming languages, and so on and so forth.
Part of the “worst-case scenario” thinking here is that we are assuming that the recruiter does not know the meaning of the questions that they themselves are asking. That means you will also have to come prepared to talk about these things as much as you are prepared to actually code how they would work.
Code Challenges as an Employer ➡️
Another popular way of gauging an employee’s skills is by issuing a coding challenge. These can come from templates online, or from your own project. Using problems from your own project as a coding challenge can benefit you greatly, as it compensates you for the time you spent on the employee by extracting a bit of labour out of them before you even have to pay them.
When you do a coding challenge, do not look too closely at the means that they take to get there, but focus on the ends. If they can make a program that does what it needs to do, then that is good even if their methodology is sloppy. Their methodology can be fixed with time, but their ability cannot be fixed.
This does mean, however, that you need a pretty clear idea of what you want them to do in order for you to issue a coding challenge. This can seem contradictory; if you know what they would need to do, why do you need them to do it? But anyone who has worked in programming knows that there is a world of difference between knowing the solution to a problem and knowing how to solve it.
Code Challenges as an Employee ➡️
One of the most common things employers will do to test people’s coding abilities is by providing them with coding challenges, sometimes straight from the project, they will be working on. Even better for them, it provides the employer with a little bit of free work on their project. But how do you prepare for such things?
Well, the answer is that you do not have to do much. In fact, coding challenges can be far easier than interviews, and in fact, will often come before an interview. The reason for this is that coding challenges can basically be treated exactly like normal programming work.
You do not have to write innovative or impressively convoluted code. In fact, it is better if it is simple enough for you to explain. That means, like all coding, you are totally within your rights to Google an answer. Some people will be apprehensive about this, but everyone in the industry knows it works.
Keep your code as clean as possible and solve the problem they hand to you as unambiguously as possible. There is a good chance that you will be given a problem, but not told very clearly what kind of solution the employer wants. In that case, the more certain your solution, the better your solution.
This is simply because if you solve the heck out of the wrong problem, then that shows much more skill than if you split the difference between three different solutions.
Conclusion
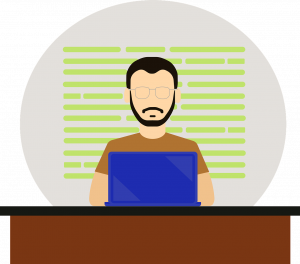
The programming industry is an interesting one. There are thousands of job listings and as many if not more employees looking for work. But it is less common than one would think for the two to find each other.
The job interview is the moment where they can—but only if they know what to look for.
This guide should help you break down that barrier. Whether you are an employer looking to scope out the next master programmer, or a common JavaScript coder just looking for a position on their first team. The important thing is that you keep an open mind and evaluate each other fairly.
And, as always, have a clear idea of what you are looking to get done together.
You Might Also Like: